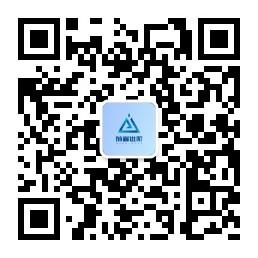
useThrottleFn
作用
用来处理函数节流的 Hook。
原理
通过调用
lodash
的throttle
方法来实现的。
源码
ts
import { throttle } from 'lodash-es'
import { useMemo } from 'react'
import useLatest from '../useLatest'
import type { ThrottleOptions } from '../useThrottle/throttleOptions'
import useUnmount from '../useUnmount'
import { isFunction } from '../utils'
import isDev from '../utils/isDev'
type noop = (...args: any[]) => any
function useThrottleFn<T extends noop>(fn: T, options?: ThrottleOptions) {
if (isDev) {
if (!isFunction(fn)) {
console.error(`useThrottleFn expected parameter is a function, got ${typeof fn}`)
}
}
// 缓存最新的 fn
const fnRef = useLatest(fn)
// 默认 1000ms
const wait = options?.wait ?? 1000
// 通过 useMemo 来缓存 throttle 函数
const throttled = useMemo(
() =>
throttle(
(...args: Parameters<T>): ReturnType<T> => {
return fnRef.current(...args)
},
wait,
options
),
[]
)
// 组件卸载时,取消节流
useUnmount(() => {
throttled.cancel()
})
return {
// 返回节流函数
run: throttled,
// 返回取消节流函数
cancel: throttled.cancel,
// 立即执行函数
flush: throttled.flush
}
}
export default useThrottleFn