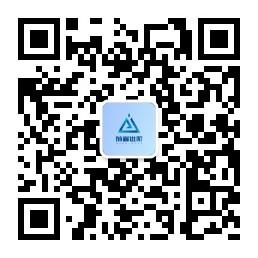
useDebounceFn
作用
用来处理防抖函数的 Hook
原理
实现原理主要是调用
lodash
的debounce
方法。
源码
ts
import { debounce } from '../utils/lodash-polyfill'
import { useMemo } from 'react'
import type { DebounceOptions } from '../useDebounce/debounceOptions'
import useLatest from '../useLatest'
import useUnmount from '../useUnmount'
import { isFunction } from '../utils'
import isDev from '../utils/isDev'
type noop = (...args: any[]) => any
function useDebounceFn<T extends noop>(fn: T, options?: DebounceOptions) {
if (isDev) {
if (!isFunction(fn)) {
console.error(`useDebounceFn expected parameter is a function, got ${typeof fn}`)
}
}
const fnRef = useLatest(fn)
// 默认延迟时间为 1000ms
const wait = options?.wait ?? 1000
const debounced = useMemo(
() =>
// 调用 lodash 的 debounce 方法
debounce(
(...args: Parameters<T>): ReturnType<T> => {
return fnRef.current(...args)
},
wait,
options,
),
[],
)
// 组件卸载时取消防抖
useUnmount(() => {
debounced.cancel()
})
return {
// 返回防抖函数
run: debounced,
// 返回取消防抖的函数
cancel: debounced.cancel,
// 返回立即执行的函数
flush: debounced.flush,
}
}
export default useDebounceFn