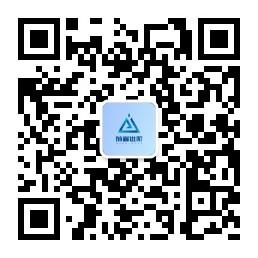
useCookieState
作用
一个可以将状态存储在 Cookie 中的 Hook
原理
通过 js-cookie 来对 Cookie 进行操作
源码
ts
import Cookies from 'js-cookie'
import { useState } from 'react'
// 持久化函数hook
import useMemoizedFn from '../useMemoizedFn'
import { isFunction, isString } from '../utils'
export type State = string | undefined
export interface Options extends Cookies.CookieAttributes {
defaultValue?: State | (() => State)
}
function useCookieState(cookieKey: string, options: Options = {}) {
const [state, setState] = useState<State>(() => {
// 从 cookie 中获取值
const cookieValue = Cookies.get(cookieKey)
// 如果 cookie 中有值,且为字符串,就返回 cookie 中的值
if (isString(cookieValue)) return cookieValue
// 如果 cookie 中没有值,且 defaultValue 是函数,就返回函数的执行结果
if (isFunction(options.defaultValue)) {
return options.defaultValue()
}
// 如果 cookie 中没有值,且 defaultValue 不是函数,就返回 defaultValue
return options.defaultValue
})
// 设置cookie值
const updateState = useMemoizedFn(
(
// 新的值
newValue: State | ((prevState: State) => State),
// 新的cookie配置
newOptions: Cookies.CookieAttributes = {}
) => {
// 合并配置,并结构出默认值
const { defaultValue, ...restOptions } = { ...options, ...newOptions }
// 如果新的值是函数,就执行函数,否则就是新的值
const value = isFunction(newValue) ? newValue(state) : newValue
// 更新状态
setState(value)
// 如果新的值是 undefined,就删除 cookie,否则就设置 cookie
if (value === undefined) {
Cookies.remove(cookieKey)
} else {
Cookies.set(cookieKey, value, restOptions)
}
}
)
// 返回状态和更新状态的函数
return [state, updateState] as const
}
export default useCookieState