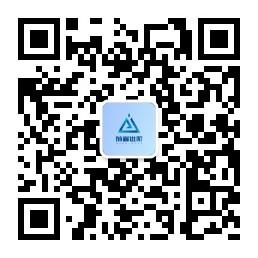
优化
打包速度
mode 可以设置 development、production
没有设置的话 4.0 会默认为 production,这个模式下会进行 tree shaking 和 uglifyjs
缩小文件的搜索范围
- alias:当我们代码中出现 import 'vue',webpack 会采用向上递归搜索的方式去 node_modules 下找。为了减少搜索范围我们可以直接告诉 webpack 去哪个路径去找,也就是别名
- include exclude 同样配置也可以减少 webpack loader 的搜索转换时间
- noParse 回去解析一个包的依赖的其他的包,告诉 webpack 不必解析
- extensions webpack 会根据 extensions 定义的后缀查找
使用 Happy 开启多进程 Loader 转换
javascript
npm i -D happypack
javascript
const HappPack = require('happypack')
const os = require('os')
const happyThreadPool = HappyPack.ThreadPool({ size: os.cpus().length })
module.exports = {
module: {
rules: [
{
test: /\.js$/,
use: [
{
loader: 'happypack/loader?id=happyBabel'
}
],
exclude: /node_moudules/
}
],
plugin: [
new HappyPack({
id: 'happyBabel',
loaders: [
{
loader: 'babel-loader',
options: {
presets: [['@babel/preset-env']],
cacheDirectory: true
}
}
],
threadPool: happyThreadPool
})
]
}
}
使用 webpack-parallel-uglify-plugin 优化压缩时间
javascript
npm i -D webpack-parallel-uglify-plugin
javascript
const ParallelUglifyPlugin = require('webpack-parallel-uglify-plugin')
module.exports = {
optimization: {
minimizer: [
new ParallelUglifyPlugin({
cacheDir: '.cache/',
uglifyJS: {
output: {
comments: false,
beautify: false
},
compress: {
drop_console: true,
collapse_vars: true,
reduce_vars: true
}
}
})
]
}
}
抽离第三方模块 使用 webpack 内置的 DllPlugin DllReferencePlugin 进行抽离,新建webpack.dll.config.js
javascript
// webpack.dll.config.js
const path = require('path')
const webpack = require('webpack')
module.exports = {
// 你想要打包的模块的数组
entry: {
vendor: ['vue', 'element-ui']
},
output: {
path: path.resolve(__dirname, 'static/js'), // 打包后文件输出的位置
filename: '[name].dll.js',
library: '[name]_library'
// 这里需要和webpack.DllPlugin中的`name: '[name]_library',`保持一致。
},
plugins: [
new webpack.DllPlugin({
path: path.resolve(__dirname, '[name]-manifest.json'),
name: '[name]_library',
context: __dirname
})
]
}
javascript
"dll": "webpack --config build/webpack.dll.config.js"
在 webpack.config.js 中增加以下代码
javascript
module.exports = {
plugins: [
new webpack.DllReferencePlugin({
context: __dirname,
manifest: require('./vendor-manifest.json')
}),
new CopyWebpackPlugin([
// 拷贝生成的文件到dist目录 这样每次不必手动去cv
{ from: 'static', to: 'static' }
])
]
}
执行
javascript
npm run dll
配置缓存
javascript
npm i -D cache-loader
javascript
module.exports = {
module: {
rules: [
{
test: /\.ext$/,
use: ['cache-loader', ...loaders],
include: path.resolve(__dirname, 'src')
}
]
}
}
优化打包体积
使用 webpack-bundle-analyzer 将打包后的直观显示
javascript
npm i -D webpack-bundle-analyer
javascript
const BundleAnalyzerPlugin = require(`webpack-bundle-analyzar`).BundleAnalyzerPlugin
module.exports = {
plugins: [
new BundleAnalyzerPlugin({
analyzerHost: '127.0.0.1',
analyzerPort: 8889
})
]
}
然后配置 script 命令
javascript
"analyz": "NODE_ENV=production npm_config_report=true npm run build"
externals
javascript
module.exports = {
//...
externals: {
jquery: 'jQuery'
}
}
tree-shaking